Calculating sums and aggregating data is a common task for Mongo users looking to derive insights from their data.
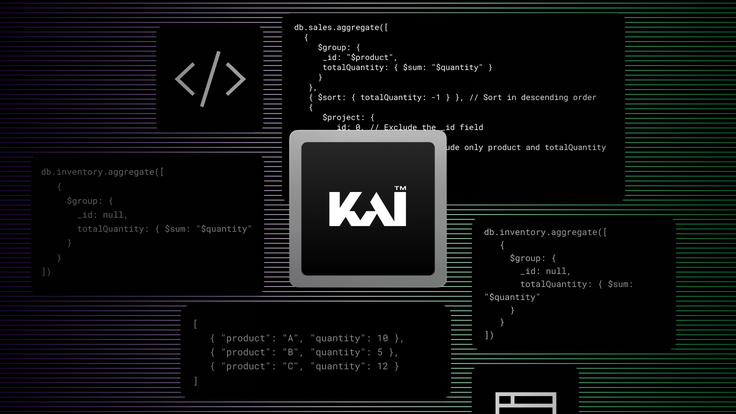
Luckily, MongoDB® Query Language (MQL) provides some built-in operators to help with this task. When calculating a sum based on fields within your data, the $sum operator is the go-to tool for such calculations, allowing users to compute the collective sum of numeric values within a collection.
In this blog, we will look at a comprehensive overview of the $sum operator, including examples of how to use the $sum operator in MongoDB. You’ll learn how it can be applied in various aggregation stages like $group, $project and $addFields to perform aggregations, handle missing fields and ignore non-numeric values. By the end of this guide, you’ll have a solid grasp of how to leverage the $sum operator to perform advanced calculations and optimizations in your MongoDB queries.
Understanding the $sum operator
The $sum operator in MongoDB is the primary tool developers can leverage to calculate the sum of numeric values. Whether you need to calculate the total sales for a specific product, determine the total quantity of items in your inventory or even tally up scores in a gaming application, the functionality within the $sum operator has you covered.
Here's a breakdown of what makes $sum so useful:
Focus on numbers. It focuses solely on numeric values within your documents, automatically handling and ignoring non-numeric data. This ensures your calculations are accurate, reliable and easy to run.
Handles missing data. If it encounters a document where the field you're summing doesn't exist, it treats it as 0. This prevents errors and ensures smooth calculations even with incomplete data.
Versatile application. $sum is incredibly versatile. It can be used within different stages of the MongoDB aggregation pipeline, including $group, $project and $addFields, allowing you to perform complex calculations and aggregations.
Syntax and behavior of $sum
Now that we know what the $sum operator can do let's take a more in-depth look at how it works and behaves. The $sum operator in MongoDB is incredibly straightforward, but understanding its syntax and behavior is key to using it for its full potential. In its simplest form, here is what the syntax looks like to use the $sum operator:
1
{ $sum: <expression> }
The <expression> within $sum is the heart of the operation. It instructs the query engine on what values to add up. This expression can be:
A field path. This is the most common scenario. You provide the path to the field containing the numeric values you want to sum. For example, "$sales" would sum up all the values in the "sales" field across your documents.
A constant. You can directly specify a constant numeric value. This is useful when adding a fixed value to a calculated sum.
An expression. $sum can handle more complex scenarios where you must calculate a value before summing it, like multiplying two fields within the expression before summing the result.
As we previously touched on, there are a few key behaviors that the $sum operator operates on that you should be aware of as you begin to use it. These include:
Numeric focus. $sum is designed to work with numbers. It automatically ignores non-numeric values it encounters, ensuring that your calculations remain accurate.
Graceful handling of missing fields. If $sum encounters a document where the specified field doesn't exist, it doesn't throw an error. Instead, it treats the missing field as 0, providing a robust solution for real-world data with inconsistencies.
Zero for non-numeric or null values. If all the values encountered by $sum are non-numeric or null, it returns 0. This consistent behavior helps prevent unexpected results.
Using the $sum operator
Next, what better way to understand the syntax and behaviors of the $sum operator than to look at some realistic examples of how it can be used? In the following examples, we will touch on some common use cases for the $sum operator.
Calculating the sum of a field across documents
First, we will look at the most fundamental use of $sum. Much of the time, developers use the $sum operator to calculate the total sum of a specific field across all documents in a collection. This gives you a single, aggregated value representing the total.
To show how this would work, imagine you have a collection called "inventory" tracking products and their quantities:
1
[2
{ "product": "A", "quantity": 10 },3
{ "product": "B", "quantity": 5 },4
{ "product": "C", "quantity": 12 }5
]
Based on the following documents, to calculate the total quantity of all products, you would use $sum like this:
1
db.inventory.aggregate([2
{3
$group: {4
_id: null,5
totalQuantity: { $sum: "$quantity" }6
}7
}8
])
The output from such a query would look like this:
1
{ "_id" : null, "totalQuantity" : 27 }
In this result, we see the $sum operator is used to calculate a single total across the entire collection of products.
Grouping and summing by category
While calculating a grand total is useful, $sum comes in especially handy when calculating sums based on different categories within your data. This is where leveraging the $group stage comes even more into play.
Using the same "inventory" collection that we used in the previous example, let's say you want to find the total quantity of each product. To do this, we would run the following MQL query:
1
db.inventory.aggregate([2
{3
$group: {4
_id: "$product",5
totalQuantity: { $sum: "$quantity" }6
}7
}8
])
The result would be an aggregation which includes each product category, and the total quantity of products available within each respective category.
1
[2
{ "_id" : "A", "totalQuantity" : 10 },3
{ "_id" : "B", "totalQuantity" : 5 },4
{ "_id" : "C", "totalQuantity" : 12 }5
]
The key concept here is the $sum works in conjunction with the $group to calculate separate totals for each distinct "product" category.
Between these two examples, the main syntax and usage of the MongoDB $sum operator is covered. However, let's take a further look at some ways the operator can be used to do more advanced sum calculations as well.
Advanced sum calculations in the aggregation framework
The $sum operator isn't limited to adding existing field values. It can be used in more advanced ways within the aggregation framework to perform complex calculations and data manipulations.
SingleStore Kai™️ further enhances this flexibility by seamlessly combining MongoDB's aggregation framework with SQL. This means you can use $sum within a larger SQL query, leveraging the strengths of both paradigms for advanced analytics. For instance, you could use SQL's window functions to calculate running totals in conjunction with $sum for more sophisticated analysis.
Using $sum with expressions and computed values
One more advanced way to use $sum is to handle expressions and computed values, allowing you to perform calculations before summing the results. For example, let's say you have a "sales" collection with fields like "price" and "quantity." To calculate the total revenue for each product, you can multiply these fields within the $sum expression:
1
db.sales.aggregate([2
{3
$group: {4
_id: "$product",5
totalRevenue: { $sum: { $multiply: ["$price", "$quantity"] } }6
}7
}8
])
In this example, the pipeline first multiplies the "price" and "quantity" fields for each document and then sums up these calculated values to get the totalRevenue for each product.
Combining $sum with other stages
In addition to some of the aggregation stages we already covered in the previous examples, $sum can be combined with other aggregation stages as well. By doing this, developers can achieve more sophisticated results from their queries. Here are a few other typical stages to combine $sum with, as well as examples based on the "sales" collection we used previously:
$sort. Sort the results based on the calculated sum. For example, you could sort products by their totalRevenue in descending order to find the top-performing products.
$project. Reshape the output documents. You can use $project to include only the necessary fields, like the product name and its totalRevenue — excluding other details from the original documents.
$addFields. Add new fields to the documents based on the calculated sum. This allows you to enrich your data with derived information, like adding a "totalSales" field to each customer document that sums up all their purchases.
As an example, let's look at an aggregation query that uses $sum and two of the stages previously mentioned, $sort and $project. Here, we can see what this type of query would look like in action. The pipeline calculates the total quantity for each product, sorts them in descending order and reshapes the output to include only the product name and its corresponding total quantity.
1
db.sales.aggregate([2
{3
$group: {4
_id: "$product",5
totalQuantity: { $sum: "$quantity" }6
}7
},8
{ $sort: { totalQuantity: -1 } }, // Sort in descending order9
{10
$project: {11
_id: 0, // Exclude the _id field12
product: "$_id",13
totalQuantity: 1 // Include only product and totalQuantity14
}15
}16
])
The result of such a query would look like this:
1
[2
{ "product": "A", "totalQuantity": 205 },3
{ "product": "C", "totalQuantity": 60 },4
{ "product": "B", "totalQuantity": 75 }5
]
In essence, this query is doing the following:
$group. Groups by "product" (A, B, or C) and sums the quantities
$sort. Sorts the grouped results in descending order of totalQuantity
$project. Cleans up the output to show only the "product" and its "totalQuantity"
As this more advanced example shows, combining the MongoDB $sum operator with expressions, computed values and other aggregation stages can unlock its full potential and perform powerful data transformations and analysis within the returned result.
Optimizing $sum performance
While $sum is a powerful tool and can do lots of useful stuff, learning how to use it efficiently is essential, especially when dealing with large datasets. Here are some tips to optimize the performance of the $sum operator to ensure your MongoDB queries run smoothly.
Leverage indexes
Indexes are crucial for any database operation, and $sum is no exception. Creating indexes on the fields involved in your $sum calculations can significantly speed up query execution. Indexes allow MongoDB to quickly locate the relevant documents, reducing the time it takes to scan through the data and calculate the sum.
Choose efficient data types
Storing any numerical value using efficient data types like integers or floats can enhance performance. These data types are optimized for arithmetic operations, making $sum calculations faster. Avoid using less efficient types like NumberDecimal unless necessary.
Break down large datasets
If you're working with vast datasets, consider breaking the data into smaller subsets and aggregating the results. This can prevent $sum from becoming computationally expensive and consuming excessive resources.
Optimize the aggregation pipeline
Employ aggregation pipeline optimization techniques to improve overall performance. This includes:
Using $match early in the pipeline. By using $match as early in the pipeline as possible to filter out unnecessary documents before performing $sum calculations, you can reduce the amount of data processed by $sum, boosting performance.
Pipeline optimization. MongoDB's query optimizer can automatically optimize aggregation pipelines. To take advantage of these optimizations, ensure you're using a recent version of MongoDB or SingleStore Kai to optimize the pipeline performance.
Monitor query performance
Lastly, regularly monitor the performance of your queries to identify areas for improvement. MongoDB provides tools like the explain() method, which can provide insights into how your queries are executed and where bottlenecks might exist. Use this information to fine-tune your queries and optimize $sum performance.
While these optimization techniques can improve $sum performance within MongoDB, for truly demanding workloads and massive datasets, SingleStore Kai is a preferred option since it is heavily optimized out of the box.
Supercharge your MongoDB performance with SingleStore Kai
Is your MongoDB application struggling to keep up with demanding workloads? Are complex analytical queries, especially those using $sum, slowing you down? SingleStore Kai is here to help.
SingleStore's distributed SQL database provides a MongoDB-compatible API called SingleStore Kai that boasts significantly faster performance for analytical queries, including those using $sum. SingleStore Kai has demonstrated up to a 100x speed improvement for common MongoDB workloads in benchmark tests. Since Kai was built to seamlessly slot into existing MongoDB workflows and applications, you can use your existing MongoDB drivers and tools but experience dramatically faster performance for your applications.
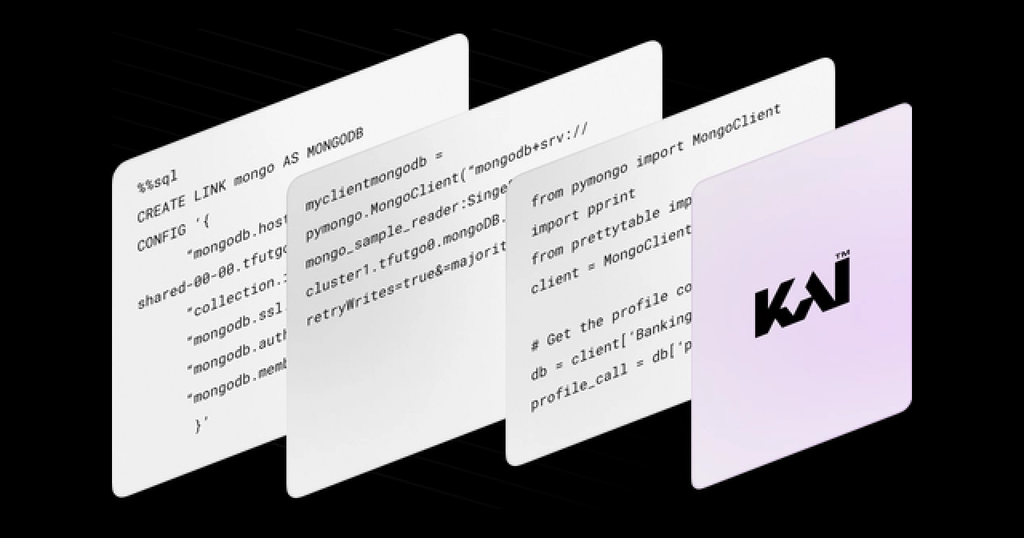
Here's why MongoDB developers should give SingleStore Kai a try:
Up to 100x faster analytical queries. See massive performance gains for your $sum operations and other aggregations, especially with large datasets.
Seamless MongoDB compatibility. Use your existing MongoDB drivers, tools and applications without code changes.
Combine the power of SQL and NoSQL. Leverage MongoDB's aggregation framework alongside SQL for ultimate flexibility.
Simplified operations. Enjoy easy deployment, effortless scaling and reduced operational overhead.
Ready to experience the difference? Try SingleStore Kai for free and unlock new levels of performance for your MongoDB applications.
Conclusion
In this blog we covered every aspect of the MongoDB $sum operator. As we learned, the $sum operator is an indispensable tool for anyone working with numeric data in MongoDB. Its versatility and efficiency make it a go-to solution for a wide range of calculations and aggregations.
Here's a recap of its key strengths:
Powerful and versatile. $sum allows you to perform essential calculations on numeric data within various stages of the aggregation framework, enabling you to generate aggregated results and gain valuable insights from your data.
Robust and reliable. It gracefully handles non-numeric values by simply ignoring them, and it treats missing fields as 0, ensuring your calculations remain accurate even with imperfect data.
Flexible and extensible. $sum can be used with expressions and computed values, allowing for more complex calculations. It can also be nested within other operators and stages, providing the flexibility to perform advanced data manipulations.
Non-destructive. As a read-only operator, $sum performs calculations without modifying the original collection, ensuring data integrity.
Whether calculating total sales, summing up inventory quantities or performing more complex aggregations, the $sum operator in MongoDB empowers developers to process and analyze their numeric data efficiently. By understanding its syntax, behavior and best practices, you can unlock its full potential and leverage it to gain valuable insights from your MongoDB collections.
If you want to take your MongoDB performance to the next level and unlock the combined power of SQL and NoSQL, sign up and give SingleStore Kai a shot today for free. Its unique architecture and optimizations can significantly accelerate your queries, including those leveraging the $sum operator. Experience the benefits of a unified platform that delivers speed, scalability, and simplicity for your MongoDB applications.