At SingleStore, we continuously explore ways to provide the best development experience through integrations with popular tools and frameworks. Today, we're excited to announce the release of the SingleStore driver for Drizzle ORM.
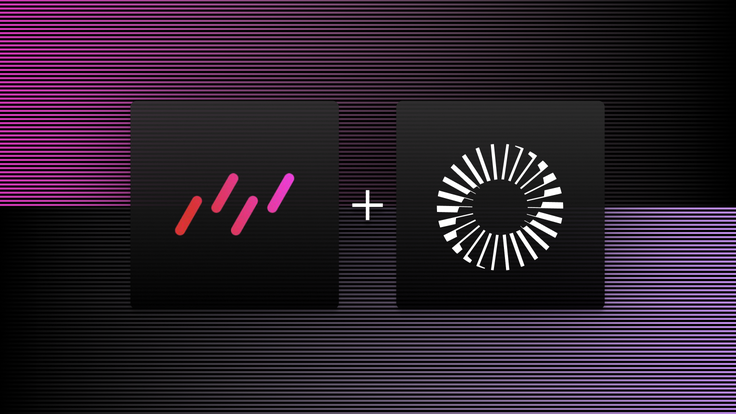
By using the SingleStore Drizzle ORM driver, you can take full control of your database schema with the flexibility of a modern, typed Object Relational Mapping (ORM). This integration combines the power of SingleStore's high-performance database with Drizzle ORM’s schema-first approach, offering seamless flexibility and type safety when developing your SingleStore applications.
To explore the full range of features and get started, visit the Drizzle website with the official documentation here, where you'll find detailed documentation and examples.
From hackathon to Drizzle integration
Drizzle ORM is a modern, lightweight, schema-first TypeScript ORM with +24k stars on GitHub. It’s the only ORM with both relational and SQL-like query APIs, providing you with the best of both worlds when it comes to accessing your relational data. Several modern databases already support Drizzle, making it a natural choice for SingleStore to join the party and offer its own integration.
The SingleStore Drizzle ORM driver started as a “lab project” for our internal summer hackathon. Each summer, SingleStore encourages everyone to collaborate and discover creative applications for its technology. After just four days, we had a prototype that was able to define SingleStore schemas and perform CRUD operations through TypeScript code.
A successful proof of concept in hand was all we needed to partner with Drizzle to deliver this driver to everyone.
Supporting Drizzle ORM
Maintaining an open-source project — especially one as essential as an ORM — isn’t easy. As developers, how many of you, who likely rely on an ORM, would be willing to pay for one? Building great software takes resources, and there are two ways to sustain it:
- Develop a business model that supports the project
- Rely on passionate volunteers
The Drizzle team has earned plenty of praise, but praise alone doesn’t pay the bills. The team is based in Ukraine, where they continue to deliver a top-notch product despite the ongoing challenges. We could’ve simply asked for SingleStore support (which we did) or contributed code ourselves (which we also did).
However, sustainability matters. Because we want to continue partnering with Drizzle and ensure their continued growth, we’re proud to become Drizzle sponsors and invite you to join us and do the same!
Getting started
Before you start
The SingleStore Drizzle driver is a TypeScript package that allows us to connect to our SingleStore database, using your favorite runtime tools like NodeJS or Bun. To use it, you need a TypeScript project. You can follow this quick tutorial to create a new one.
We will also need a SingleStore database, which we can start for free here.
Install the SingleStore driver
Using NPM
1
npm i drizzle-orm mysql22
npm i -D drizzle-kit
Using Yarn
1
yarn add drizzle-orm mysql22
yarn add -D drizzle-kit
Using PNPM
1
pnpm add drizzle-orm mysql22
pnpm add -D drizzle-kit
Using Bun
1
bun add drizzle-orm mysql22
bun add -D drizzle-kit
Connect to SingleStore
To connect to our SingleStore database, create a new file called app.ts in the root directory. It is inside this file that we are going to create a new connection to your SingleStore database. To create a new DB connection, we initialize the Drizzle client from the SingleStore driver and pass the credentials to a mysql2 connection.
1
import { drizzle } from "drizzle-orm/singlestore";2
import mysql from "mysql2/promise";3
4
const connection = await mysql.createConnection("<username>:<password>@<host>:<port>/<database>?ssl={}");5
6
const db = drizzle({ client: connection });
Or we can pass the database credentials to the mysql2 connection
1
import { drizzle } from "drizzle-orm/singlestore";2
import mysql from "mysql2/promise";3
4
const connection = await mysql.createConnection({5
host: "host",6
user: "user",7
database: "database",8
ssl: {}9
...10
});11
12
const db = drizzle({ client: connection });
Since we are using the SingleStore Portal, we can get our database credentials by selecting Deployments > Connect > “SQL IDE”.
.png?width=1024&disable=upscale&auto=webp)
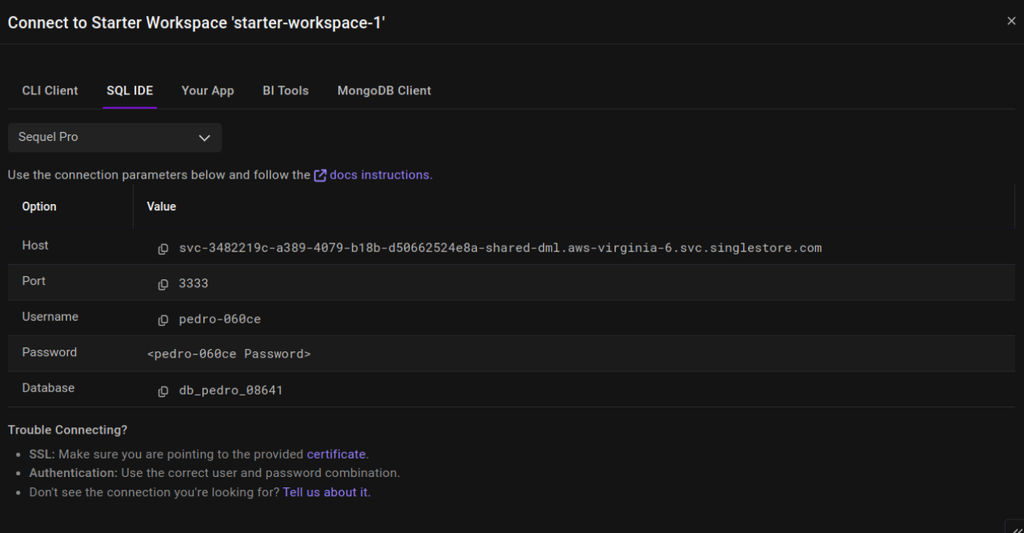
Define your database schema
The schema is the blueprint of our database. It contains information about our tables and their columns, such as their name and type. To define our database schema, we can create the schema.ts file inside the db directory.
1
📦 <project root>2
└ 📜 app.ts3
└ 📂 db4
└ 📜 schema.ts
It is possible to define the table schemas through TypeScript code with Drizzle ORM. In this example, we create the users_table
table, that contains the information about the users in our application. The users are defined by their ID (primary key), name and age.
1
import { int, text, singlestoreTable } from "drizzle-orm/singlestore-core";2
3
export const users = singlestoreTable("users_table", {4
id: bigint("id", { mode: "bigint" }).primaryKey().autoincrement(),5
name: text("name"),6
age: int("age"),7
});
To apply our schema on our SingleStore database, Drizzle needs a configuration file named drizzle.config.ts. In this file, we specify the path to the schema.ts file, and the dialect, which in our case is singlestore
. You can find more information on how to customize the drizzle.config.ts file here.
1
import { defineConfig } from "drizzle-kit";2
3
export default defineConfig({4
dialect: 'singlestore',5
schema: './db/schema.ts'6
})
For more information about the drizzle.config.ts file and migrations with Drizzle, please check this link.
Create the users table
To create the users_table
table on our SingleStore database, we need to first generate and then apply the Drizzle migrations. Migrations are snapshots of the current state of your database schema and it’s how Drizzle ORM tracks when and how the database schema changes.
To generate the migration files, you can run the following command.
1
npx drizzle-kit generate
This creates the migration file, which is an SQL file that contains the SQL statements required to create our users_table
table. Feel free to inspect the migration file generated by the Drizzle ORM.
1
📦 <project root>2
└ 📂 drizzle3
└ 📂 drizzle4
└ 📜 0000_<first-migration>.sql5
└ 📜 app.ts6
└ 📂 db7
└ 📜 schema.ts
After generating the migration files, we need to apply the migration to our database. To apply this migration, run the following command.
1
npx drizzle-kit migrate
If we go back to the SingleStore Portal and inspect our database, we will find the users_table table with the schema defined before.

Populate the database
Now that we created the users_table
table, we can create our records. We can insert them into our table using the functions insert
and values
. In the following example, we are importing the users
table to the app.ts file, and inserting five new records.
1
// app.ts2
import { users } from "./db/schema.ts"3
4
// ...5
6
const newUsers = [7
{8
name: 'John',9
age: 28,10
},11
{12
name: 'Jane',13
age: 32,14
},15
{16
name: 'Jack',17
age: 25,18
},19
{20
name: 'Jill',21
age: 24,22
},23
{24
name: 'James',25
age: 30,26
}27
]28
29
await db.insert(users).values(newUsers);
1
// Fetch all users2
const rows = await db.select().from(usersTable)3
4
// Print all users5
console.log(rows)
For a full guide, check out this example. We also encourage you to browse through the official documentation.
What's next?
The current version of the SingleStore Drizzle ORM driver offers limited features. You can define your database schema and perform queries but we also want to support more features in the future, such as column types that SingleStore supports which are not supported by other databases and row level security.
Nevertheless, these examples provide a glimpse of what’s possible with the SingleStore Drizzle ORM driver. If you want to see it in action, explore and run a basic TypeScript application example that bootstraps SingleStore and Drizzle integration, click here!
Are you ready to power your next TypeScript application with SingleStore’s unmatched speed and flexibility for OLTP and OLAP workloads? Start your free SingleStore trial today, and use the new Drizzle integration to seamlessly connect your application to the world’s fastest unified database platform.