To make SingleStore even more accessible for JavaScript and Node.js developers, we're excited to introduce a powerful new tool for developers: the @singlestore/client NPM package.
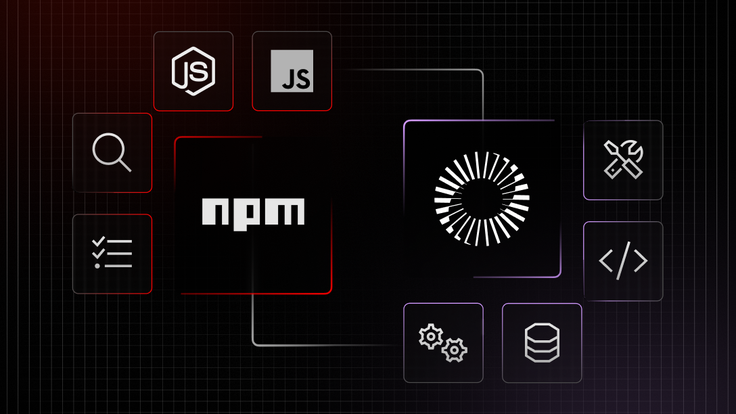
By using this package within your Node.js applications, you can simplify the integration of SingleStore's high-performance capabilities into your JavaScript applications.
Using this package lets you quickly have complete control over your data, making real-time applications more accessible to build and maintain. With a clean and intuitive API, @singlestore/client allows you to effortlessly manage organizations, workspace groups, workspaces, databases, tables, columns, scheduled jobs and other critical components of the SingleStore Management API. This gives developers a more effortless way to leverage the full potential of SingleStore in their projects. To explore the complete list of features, visit the NPM page — which includes a full table of contents for the package's functionality.
Initialization
To start using the @singlestore/client package, you must install it then initialize the SingleStore client. To install the package into your project using npm
, you will need to run the following npm install
command in your terminal:
npm install @singlestore/client
Once the package is installed, there are several ways to initialize it, depending on your goals. Let’s walk through each option step by step.
Sometimes, developers may not require the more extended functionalities available within the package (including AI and Management API capabilities). In these cases, the SingleStore client can be used to connect to the database and run DDL, DML and DQL statements. To initialize the client and facilitate this type of connectivity, you can simply create an instance of the class without any parameters. Here is an example:
import { SingleStoreClient } from "@singlestore/client";const client = new SingleStoreClient();
You may also want to leverage the AI functionality that is exposed within SingleStore. In this case, you need to create an instance of the AI class from the @singlestore/ai
package and specify the ai parameter when initializing the SingleStoreClient
. Similar to the previous example of database-only connectivity, here is how you would also initialize the package with AI functionality enabled.
import { AI } from "@singlestore/ai";import { SingleStoreClient } from "@singlestore/client";const ai = new AI({ openAIApiKey: "<OPENAI_API_KEY>" });const client = new SingleStoreClient({ ai });
It’s important to note the OpenAI model implements AI functionality by default. However, the client can also support other platforms and models. To use other platforms, you can override the default classes by following this guide.
In cases where you want to also access functionality exposed via the SingleStore Management API, you can do this through the client as well. To initialize the client with this functionality available, you should specify the apiKey
parameter when initializing the SingleStoreClient
. An example of this can be seen here.
import { SingleStoreClient } from "@singlestore/client";const client = new SingleStoreClient({ apiKey: "<SINGLESTORE_API_KEY>" });
By leveraging the client for database interactions, you can use both the AI and Management API capabilities within a single instance of the SingleStoreClient
class, instead of instantiating an instance for each use. The result is a unified interface for all your needs when it comes to using SingleStore in your Node.js applications.
Basic usage example
To look at a more concrete example of how to use the SingleStore client, let's walk through the code of an application that is using the client ’s functionalities. In the following application you can see the process of creating an instance of the SingleStoreClient
class, establishing a connection to the database, performing CRUD operations, performing a vector search and creating a chat completion.
Firstly, our application must import the @singlestore/ai and @singlestore/client dependencies. Since we plan to use the AI functionality, we need to initialize an instance of the SingleStore AI object and initialize the SingleStoreClient with the ai parameter, passing our AI client instance into it.
import { AI } from "@singlestore/ai";import { SingleStoreClient } from "@singlestore/client";const ai = new AI({ openAIApiKey: "<OPENAI_API_KEY>" });const client = new SingleStoreClient({ ai });
Next, we need to establish a connection to the database. For this we will need to supply some workspace details including the host
, user
, password
, and port
. Then, once the connection object is defined we will use it to connect to the database, storing the connection in the database
database variable.
const connection = client.connect({host: "<WORKSPACE_HOST>",user: "<WORKSPACE_USER>",password: "<WORKSPACE_PASSWORD>",port: <WORKSPACE_PORT>});const database = connection.database.use<DatabaseSchema>("<DATABASE_NAME>");
After we’ve established the connectivity to our SingleStore instance, we can then begin to interact with the database. In this example, let's assume that our database contains a table with users, and we need to perform CRUD operations on it. First, we can use the database object to create a variable that references the users table.
const usersTable = database.table.use("users")
Then, we can proceed to perform different CRUD operations through the interface exposed by the table object. Here are a few examples of CRUD operations being done through the usersTable
object we created.
// Get all usersconst users = await usersTable.find();// Get users by a conditionconst users = await usersTable.find({where: {name: "John",hobby: { in: ["music", "drawing"] },},});// Insert a userawait usersTable.insert({name: "John",hobby: "cycling",});// Update a userawait usersTable.update({ hobby: "driving" }, { id: "<ID>" });// Delete a userawait usersTable.delete({id: "<ID>"})
If you’re looking for additional functionality, there are several other methods exposed through the SingleStore client for working with tables. For a complete list, you can check out the reference here.
At SingleStore, we have also heavily focused on providing users with access to cutting-edge AI capabilities. Many of our users are interested in utilizing AI functionality, like vector search or creating chat completions. The SingleStore Client provides methods to perform these tasks out of the box. To use the client to bring in these functionalities, let's start by looking at a schema that demonstrates how to use the vector search feature exposed in the client.
const rows = await table.vectorSearch({prompt: "<PROMPT>",vectorColumn: "<VECTOR_COLUMN_NAME>",},{select: ["<COLUMN_NAME>"], // Optionaljoin: [{type: "FULL", // Supported values: "INNER" | "LEFT" | "RIGHT" | "FULL"table: "<JOIN_TABLE_NAME>",as: "<JOIN_TABLE_AS>",on: ["<COLUMN_NAME>","=", // Supported values: "=" | "<" | ">" | "<=" | ">=" | "!=""<JOIN_COLUMN_NAME>",],},], // Optionalwhere: { columnName: "<COLUMN_VALUE>" }, // OptionalgroupBy: ["<COLUMN_NAME>"], // OptionalorderBy: {columnName: "asc", // Supported values: "asc" | "desc"}, // Optionallimit: 10, // Optionaloffset: 10, // Optional}, // Optional);
As you can see from the example, to perform a vector search, you need to declare the prompt
and vectorColumn
parameters and optionally set a condition for the search. After running this vector search query through the SingleStore client, the result contains rows from the table that match the search criteria, and can be used within the Node.js application.
Lastly, let's look at how to create a chat completion within the SingleStore client. To do this, we can use our connection to the database and call the createChatCompletion
method to dispatch the chat completion query. Here is an example of how this can be done.
const chatCompletion = await table.createChatCompletion({model: "<MODEL_NAME>", // Optionalprompt: "<PROMPT>",systemRole: "<SYSTEM_ROLE>", // OptionalvectorColumn: "<VECTOR_COLUMN_NAME>",stream: false,temperature: 0, // Optional},{select: ["<COLUMN_NAME>"], // Optionalwhere: { columnName: "<COLUMN_VALUE>" }, // Optionallimit: 1, // Optional}, // Optional);
In the result returned from the chat completion query, you will receive a chatCompletion
object containing a content
field containing the returned data. This can then be used within your application. Additionally, you can also utilize streaming and handle stream chunks if you require this type of functionality. For the specifics on how to implement this, refer to this documentation for more details.
Try it for yourself!
These were simple examples showcasing some of the available functionality of this package. You can also explore and run a basic Next.js application example that uses the SingleStore Client by following this link. Are you looking to unlock the functionalities contained within SingleStore for your next Node.js application? Start your free SingleStore trial and use the @singlestore/client
package in your Node.js application to easily integrate your application with the world’s fastest and more flexible database for OTLP and OLAP workloads!