Need to count documents in your MongoDB collections? The aggregation framework is your go-to tool; the $count stage is essential for this task.
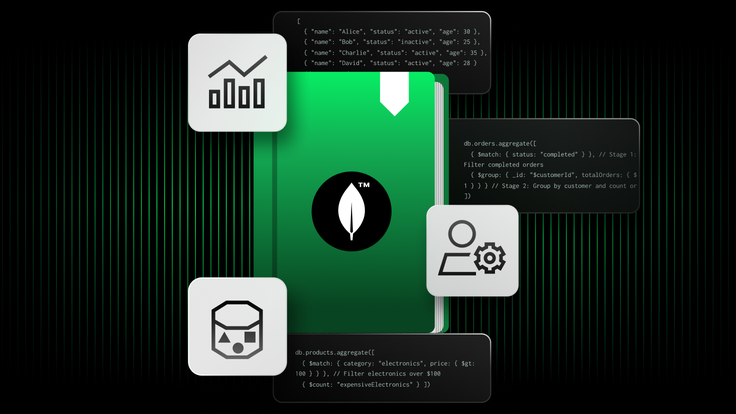
This guide will explain everything you need to know about using $count effectively within your applications and queries using Mongo Query Language. We'll cover the basics, show you practical examples and even share tips for optimizing performance. Let's look at how the MongoDB aggregation framework works before hopping into the specifics of $count.
Understanding MongoDB aggregation
MongoDB's aggregation framework is a key tool for processing and analyzing data within collections. It allows you to perform complex operations on documents by passing them through a series of stages, which form an aggregation pipeline. Each stage performs a specific action, like filtering ($match), sorting ($sort), grouping ($group), reshaping ($project) and more.
This pipeline approach enables advanced data manipulation and analysis. You can calculate statistics, group and aggregate data, and transform documents to meet specific requirements. When it comes to operations like the ones that can be implemented using $count, aggregations offer greater flexibility and efficiency compared to simpler methods like estimatedDocumentCount() and count() — especially when dealing with large datasets or complex queries.
Understanding the aggregation framework will help you better use it and unlock MongoDB's full potential for data processing and analysis. Next, let's explore the aggregation pipeline further and cover some key concepts using examples.
The aggregation pipeline
The core of MongoDB's aggregation framework is the pipeline. As mentioned in the previous section, it's a sequence of stages where each stage transforms the input documents passing through it. Think of it as a series of data processing steps, where the output of one stage becomes the input for the next.
Here's a simple example to illustrate the concept:
db.orders.aggregate([{ $match: { status: "completed" } }, // Stage 1: Filter completed orders{ $group: { _id: "$customerId", totalOrders: { $sum: 1 } } } // Stage 2: Group by customer and count orders])
In this pipeline, the $match stage filters the orders collection to select only documents where the status field is "completed". Then, the $group stage groups the filtered documents by the customerId field and uses $sum to count the orders for each customer.
This pipeline takes a collection of orders and outputs a new collection where each document represents a customer and their total number of completed orders.
Based on this example, you can get a rough idea of how the pipeline works. Key characteristics of the aggregation pipeline:
Sequential execution. Stages execute in the order they appear in the pipeline
Data transformation. Each stage transforms the data, refining it towards the desired outcome
Flexibility. You can combine different stages to perform complex operations
Modularity. You can add or remove stages to adjust the pipeline's behavior
By understanding the pipeline concept, you can effectively chain different aggregation stages — including $count — to perform powerful data processing and analysis in MongoDB. Of course, even though the aggregation pipeline has many benefits and flexibility, there are also some nuances to be aware of.
Aggregation stage limits
While MongoDB's aggregation framework is a powerful tool for analyzing data, it's essential to be aware of certain limitations that can affect performance and resource usage. Much of the time, these limitations come in the form of memory limits. These include:
Per-stage memory. Each stage in the aggregation pipeline has a memory limit. If a stage exceeds this limit during execution, the database will return an error. This limit helps prevent individual stages from consuming excessive resources
Output document size. The documents the aggregation query returns are also subject to a size limit. Exceeding this limit on a single document will result in an error
Want to surpass these limits? Use SingleStore Kai™️ to break out of the limitations set by a typical MongoDB deployment. As a distributed SQL database with a MongoDB-compatible API, SingleStore offers higher limits for memory consumption and document size, allowing for more complex operations and handling of larger datasets within aggregations.
To reduce the chances these limitations will affect your operations, you can use a few of the following best practices to reduce issues due to exceeding memory limits. These include:
Monitor memory usage. When working with large datasets or complex pipelines, it's a good practice to monitor each stage's memory usage to identify potential bottlenecks and optimize queries
Optimize pipeline. Techniques like using $match early in the pipeline can reduce the number of documents processed by subsequent stages, improving efficiency
Utilize indexes. Appropriate indexes on the fields used in your aggregation queries can significantly improve performance and reduce memory consumption
By being mindful of these limitations and best practices and considering alternatives like SingleStore Kai, you can ensure your aggregation pipelines run efficiently and effectively regardless of complexity. Now that we are familiar with the inner workings of aggregations, let's take a closer look at incorporating $count into our pipelines.
Using the $count stage
As you know, MongoDB offers many different stages to help you compute and transform your data through aggregations. Of the available stages, the $count stage in MongoDB's aggregation pipeline is your go-to for operations that require counting documents. It lets you determine how many documents make it through the previous stages of your pipeline.
When it comes to the syntax of using this stage, it's extremely simple:
{ $count: "<string>" }
Within this syntax, the <string> variable represents the name of the field where the count will be stored.
What does this syntax look like in practice? Let's say you have a users collection that looks like this:
[{ "name": "Alice", "status": "active", "age": 30 },{ "name": "Bob", "status": "inactive", "age": 25 },{ "name": "Charlie", "status": "active", "age": 35 },{ "name": "David", "status": "active", "age": 28 }// ... more documents]
Based on this data, let's say that you want to count how many users are labelled as "active". Here's how you could use $count in the pipeline to calculate and get the result:
db.users.aggregate([{ $match: { status: "active" } }, // Filter for active users{ $count: "activeUsers" } // Count the filtered documents])
Running this aggregation would return the following output:
{ "activeUsers" : 3 }
This result of the aggregation being equal to 3 is because Alice, Charlie and David have a status equal to "active".
As you can tell from this example, there are a few key considerations to remember when using the $count stage. First, you'll want to be aware of the stage's placement. Generally, this means putting $count at the end of your pipeline to count all the documents that passed through the previous stages. Secondly, for those familiar with other methods for counting documents, you can use $group with $sum: 1 to achieve a functionally equivalent result; however, $count is more concise and readable for certain tasks. In general, the $count stage is handy for:
Counting filtered documents. Find how many documents meet specific criteria
Counting grouped documents. Count documents in each group after using the $group stage
Generating metrics. Calculate counts for reports and dashboards
Let's explore some more examples and use cases where $count can be a helpful addition to your aggregations.
Working with specified values
Like most stages, the $count stage works best when combined with other stages in the aggregation pipeline. This allows you to count documents based on specific criteria and perform more complex analyses. In this next section, let's look at how $count can be combined with stages including $match and $group to perform calculations.
Filtering with $match
The $match stage is used to filter documents based on specified conditions. Using $match before $count, you can count only the documents that meet your criteria. For example, let's say you have the following data set you've inserted into your database:
db.products.insertMany([{ "_id": 1, "productName": "Laptop", "category": "electronics", "price": 1200 },{ "_id": 2, "productName": "Smartphone", "category": "electronics", "price": 800 },{ "_id": 3, "productName": "Desk Chair", "category": "furniture", "price": 150 },{ "_id": 4, "productName": "Headphones", "category": "electronics", "price": 100 },{ "_id": 5, "productName": "Desk", "category": "furniture", "price": 200 }])
We could then use $match to filter the results to only those entries that are "electronics" and have a price that is greater than $100. After this first stage, we could then apply the $count stage to retrieve the count of those items, which would be considered "expensiveElectronics". Here's what that query would look like:
db.products.aggregate([{ $match: { category: "electronics", price: { $gt: 100 } } }, // Filter electronics over $100{ $count: "expensiveElectronics" }])
The output from these two stages would look like this:
[ { expensiveElectronics: 2 } ]
Grouping with $group
The $group stage groups documents based on a specified field and allows you to perform aggregations within each group. You can use $count after $group to count the documents in each group. For example, let's say you created the following data set loaded into your Mongo instance:
db.sales.insertMany([{ "_id": 1, "customerId": 1, "amount": 1200 },{ "_id": 2, "customerId": 2, "amount": 800 },{ "_id": 3, "customerId": 1, "amount": 150 },{ "_id": 4, "customerId": 4, "amount": 200 },{ "_id": 5, "customerId": 3, "amount": 100 }])
Within this data set, let's take a look at how we could use $group and $count to group all of the purchases by $customerId, then count how many customers have made a purchase. This is what this query may look like:
db.sales.aggregate([{ $group: { _id: "$customerId" } }, // Group by customerId to get unique customers{ $count: "customerCount" } // Count the number of unique customers])
In the following example, the returned result from this query shows the total count of all customers that made a purchase:
[ { customerCount: 4 } ]
Although these examples are simple, they help show how $count can be used within aggregation pipelines to count documents. Combining $count with other stages like $match and $group allows you to perform more sophisticated analysis and extract valuable insights from the data you have stored within your MongoDB tables.
Performance considerations
When working with aggregation pipelines — especially those involving large datasets or complex operations — performance should be something to be aware of. Similar to optimizing queries in the SQL realm, optimizing pipelines can significantly improve query execution speed and reduce resource consumption when they are executed. Here are some key performance considerations to remember when creating an aggregation query:
Stage order. The order of stages in your pipeline can impact performance. For instance, filtering with $match early on can reduce the number of documents processed by subsequent stages, improving overall efficiency
Indexes. Ensure you have appropriate indexes on the fields used in your aggregation queries. Indexes can dramatically speed up data retrieval, and reduce the work the database needs to perform
Data cardinality. High cardinality in grouping fields (fields with many distinct values) can lead to increased memory usage and slower performance for $group operations. If dealing with high-cardinality data, consider alternative approaches or optimization techniques, like breaking down data into smaller subsets, using pre-aggregated results or leveraging indexing strategies that target specific query patterns
Pipeline complexity. Avoid overly complex pipelines with numerous stages or complex expressions. Break down complex operations into smaller, more manageable stages to improve readability and performance
Monitoring and profiling. Utilize MongoDB's profiling tools to monitor the performance of your aggregation queries. This can help identify bottlenecks and areas for optimization
If you're facing performance challenges with MongoDB aggregations, SingleStore Kai offers a great alternative. It allows you to use your existing MongoDB tool kit (like Mongoose or other tools within your applications) but gives the benefit of blazing-fast NoSQL query execution, as well as SQL. Its distributed architecture, columnar storage and advanced query optimizer can significantly accelerate aggregation pipelines, even for demanding workloads. SingleStore Kai is designed to handle large datasets and complex queries efficiently out-of-the-box, offering superior performance compared to MongoDB in many scenarios.
In the next section, we'll explore how SingleStore Kai specifically addresses performance bottlenecks and enhance the efficiency of your MongoDB aggregations.
Supercharge your MongoDB $count performance with SingleStore Kai
Is your MongoDB application struggling to keep up with demanding workloads? Are your $count aggregations slowing you down, especially those within complex pipelines or operating on large datasets? SingleStore Kai is here to help.
SingleStore Kai builds on SingleStore's distributed SQL database with a MongoDB-compatible API that delivers blazing-fast performance for analytical queries, including those using $count. In benchmark tests, SingleStore Kai has demonstrated up to a 100x speed improvement for common MongoDB workloads. Because it is designed to integrate with your existing MongoDB workflows seamlessly, you can use your current MongoDB drivers and tools while experiencing dramatically faster performance.
Here's why MongoDB developers should give SingleStore Kai a try:
Up to 100x faster analytical queries. See massive performance gains for your $count operations and complex aggregations, especially with large datasets
Seamless MongoDB compatibility. Use existing MongoDB drivers, tools and applications without modifying any code
Combine the power of SQL and NoSQL. For ultimate flexibility, leverage MongoDB's aggregation framework — including $count — alongside the power and familiarity of SQL
Simplified operations. Enjoy easy deployment, effortless scaling and reduced operational overhead
Ready to experience the difference? Try SingleStore Kai for free and unlock new levels of performance for your MongoDB applications.
Try SingleStore Kai
The $count stage in MongoDB's aggregation framework is valuable for efficiently counting documents within your collections. Whether you need to count all documents, filter based on specific criteria or count within grouped data, $count provides a simple interface and effective solution for these calculations. By understanding its syntax, usage and performance considerations, you can leverage this stage to gain valuable insights from your data.
And remember, if you're facing performance challenges with MongoDB aggregations, SingleStore Kai is a great alternative that is directly compatible with your existing MongoDB tooling. Its distributed architecture and optimizations can significantly accelerate your $count operations and unlock new levels of performance for your MongoDB applications. Try SingleStore Kai free, and see just how performant it is for yourself compared to standard MongoDB instances.