When it comes to storing data in MongoDB®, arrays are a common way to store data collections. Just as there are many ways to work with JSON arrays in many languages, Mongo Query Language also has the same nuances.
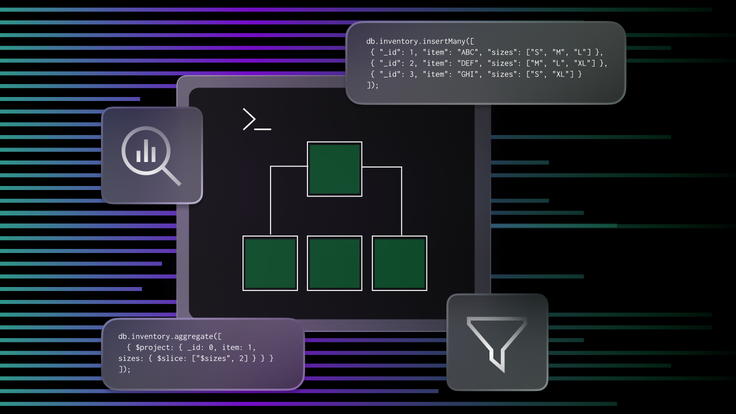
This means that querying arrays effectively in Mongo can sometimes feel slightly more complex than we would like. Luckily, we've thrown together this extensive guide to give you everything you need to query and filter elements stored within arrays in MongoDB effectively.
This guide dives deep into the practical aspects of MongoDB and how to query an array. We'll explore various methods, from simple dot notation to useful operators like $elemMatch and $all, and even touch on advanced techniques and performance optimization. Let's begin by looking at the fundamentals and understanding the basics of how to query arrays.
Understanding MongoDB array queries
As you likely know, MongoDB stores data in documents. These documents can contain arrays to hold lists of related values. When you need to find documents based on the contents of these arrays, you would use array queries. For example, let's say we have a collection called products that contains the following document within it:
{"_id": 1,"name": "Laptop","tags": ["electronics", "computer", "portable"]}
To find documents where the tags array contains the element "computer", we could query to parse the data and locate our desired document or value. Fortunately, MongoDB offers several methods for querying arrays. These methods include:
find() method. This is the primary method for querying documents in MongoDB, including those with arrays. It allows you to specify criteria for matching documents based on their field values, including array fields.
Dot notation. Access specific elements in an array by their position (index). For example, based on the preceding document, tags.0 would refer to the first element in the tags array, which happens to be ("electronics").
$elemMatch operator. This method matches documents where at least one array element is an exact match for multiple criteria. This is useful when you need to query multiple fields within an array element.
$slice operator. This is useful when retrieving a portion of an array. You can specify the starting index and the number of elements to retrieve.
$all operator. This method, similar to $elemMatch, matches documents where the array contains all specified elements. This is useful when you must ensure the array has all the values you want, regardless of their order.
Using these methods, you have the basic building blocks of querying arrays in your pocket. Understanding how these methods can be used is essential for efficiently retrieving data from arrays within your MongoDB documents.
Querying array elements
MongoDB's find() method is your primary tool for querying array elements within documents. Combined with the other methods mentioned in the last section, this will be how you find and extract data from arrays using Mongo Query Language. Depending on how complex your data and query need to be, different approaches will work better than others.
Basic matching
We can use basic matching to retrieve a document or value at the most simple level. To find documents where an array field contains at least one element with a specific value, you can use a simple filter like this:
db.collection.find({ <field>: <value> });
This query has a value for <field> and one for <value>. The <field> is the name of the array field in your documents, and <value> is the value of the element you're searching for.
For example, if you have a products collection with a tags array field, like the one seen in the insert statement here:
db.products.insertMany([{ "_id": 1, "name": "Laptop", "tags": ["electronics", "computer", "portable"] },{ "_id": 2, "name": "Tablet", "tags": ["electronics", "portable"] },{ "_id": 3, "name": "Smartphone", "tags": ["electronics", "communication"] }]);
You can find all products tagged with "computer" using the following basic query:
db.products.find({ tags: "computer" });
The result from this query would return the following data:
[{_id: 1,name: 'Laptop',tags: [ 'electronics', 'computer', 'portable' ]}]
Only the laptop entry would be returned since it is the only one out of the three documents that is tagged as a computer within the tags array.
Dot notation
If we want to be more precise, we can use dot notation to access elements within the array by their index. For example, to find products where the second element (index 1) in the tags array is "computer", you can use:
db.products.find({ "tags.1": "computer" });
The returned result would match our previous find() query:
[{_id: 1,name: 'Laptop',tags: [ 'electronics', 'computer', 'portable' ]}]
However, this would only return results where the exact term is the element within that exact array element. In some scenarios, for example, where an array is ordered or sorted, this could be quite useful and more precise.
Using query operators
You can incorporate query operators within your filter document for more complex matching. These operators allow you to specify conditions on the elements in the array field. For example, to find products with a tags array that contains an element that starts with "com", you could use the $regex operator:
db.products.find({ tags: { $regex: /^com/ } });
This query would match documents where at least one tags array element starts with "com". So this means that we would see the following result returned from the query:
[{_id: 1,name: 'Laptop',tags: [ 'electronics', 'computer', 'portable' ]},{_id: 3,name: 'Smartphone',tags: [ 'electronics', 'communication' ]}]
Both the laptop and smartphone entries have tags that contain "com", "computer" and "communication" for the smartphone.
This outlines the basic building blocks of querying arrays using Mongo Query Language. However, most use cases will be significantly more complex. For those, let's look at some more advanced querying examples.
Querying array elements with multiple values
Sometimes, you must query array elements based on multiple values or conditions. Luckily, MongoDB provides powerful operators to handle these scenarios with relative ease. In this section, we will look at a few ways this is made possible using $elemMatch, $all and $filter, and how we can combine these operators to hone in on the data we want.
$elemMatch for multiple criteria
As talked about when we first looked at various methods for querying arrays, the $elemMatch operator is crucial when you need to match documents where at least one array element satisfies multiple criteria. This ensures all the conditions are met within the same element.
For example, let's say we've inserted the following students collection into our database, which contains a grades array field:
db.students.insertMany([{ "_id": 1, "name": "Alice", "grades": [ { "course": "Math", "score": 90 }, { "course": "Science", "score": 85 } ], "favoriteSubjects": ["Math", "Science", "History"] },{ "_id": 2, "name": "Bob", "grades": [ { "course": "Math", "score": 78 }, { "course": "Science", "score": 92 } ], "favoriteSubjects": ["Math", "English", "Art"] },{ "_id": 3, "name": "Charlie", "grades": [ { "course": "History", "score": 88 }, { "course": "Music", "score": 95 } ], "favoriteSubjects": ["Science", "History", "Music"] }]);
Using $elemMatch, we can construct a query to find students who have a "Math" grade with a score greater than 80. That query would look like this:
db.students.find({ grades: { $elemMatch: { course: "Math", score: { $gt: 80 } } } });
The result of this query would be:
[{_id: 1,name: 'Alice',grades: [{ course: 'Math', score: 90 },{ course: 'Science', score: 85 }],favoriteSubjects: [ 'Math', 'Science', 'History' ]}]
This is because Alice's grade in "Math" matches the "greater than 80%" threshold we specified in the query. Even though Bob had a 92% in "Science", since it didn't meet the criteria for the course being "Math", the result does not contain Bob's entry. When looking for multi-faceted queries, $elemMatch is a great tool to understand and use.
$all for matching all values
Building up our arsenal of operators we can use in our queries, the next useful one to focus on is the $all operator. This one is useful when you need to match documents where the array contains all the specified values, regardless of their order.
Using the same data we used for the preceding example, let's imagine an example query where we want to find students with "Math" and "Science" listed as their favorite subjects. Using the students collection, the query would look like this:
db.students.find({ favoriteSubjects: { $all: ["Math", "Science"] } });
This query would only match the document with _id: 1 (Alice) because it's the only one in which the favoriteSubjects array contains both "Math" and "Science."
[{_id: 1,name: 'Alice',grades: [{ course: 'Math', score: 90 },{ course: 'Science', score: 85 }],favoriteSubjects: [ 'Math', 'Science', 'History' ]}]
Although, in this example, the order in the query is the same as the order within the array, the order of the elements in the array doesn't matter; as long as both values are present, the document will match.
Combining operators
For more targeted querying, you can even combine these operators for more complex lookups. For example, to find students with a "Math" grade over 80 and a "Science" grade over 70, you could use $elemMatch with $and operators together. An example of such a query would look like this:
db.students.find({grades: {$all: [{ $elemMatch: { course: "Math", score: { $gt: 80 } } },{ $elemMatch: { course: "Science", score: { $gt: 70 } } }]}});
The returned result from the query would look like this:
[{_id: 1,name: 'Alice',grades: [{ course: 'Math', score: 90 },{ course: 'Science', score: 85 }],favoriteSubjects: [ 'Math', 'Science', 'History' ]}]
Again, only one student in the database matches this query criteria, so we will see Alice's entry returned once again. This example shows how to combine operators to get even more specific on the data you want.
$filter in aggregation
You can leverage the $filter operator within the aggregation framework for advanced filtering and extraction of array elements. This allows you to define more complex logic and transformations on array elements beyond simply querying them.
For example, let's say you want to extract only the grades where the score is greater than or equal to 90 from the grades array in the students collection. Using an aggregation pipeline, we can do exactly this:
db.students.aggregate([{$project: {_id: 0,name: 1,topGrades: {$filter: {input: "$grades",as: "grade",cond: { $gte: ["$$grade.score", 90] }}}}}]);
Once this pipeline has been executed, you will see the following result:
[{ "name": "Alice", "topGrades": [ { "course": "Math", "score": 90 } ] },{ "name": "Bob", "topGrades": [] },{ "name": "Charlie", "topGrades": [ { "course": "Music", "score": 95 } ] }]
As you can see, all the documents returned contain a topGrades field in the output, which contains only the grades from the original grades array where the score is greater than or equal to 90. Since Bob has no grades above 90%, you see no entry in the topGrades array for him.
The $filter operator is a really powerful way to perform complex filtering and extraction of array elements within the aggregation framework. You can define custom conditions and logic to precisely control which elements are included in the output and shape the output to match exactly what you want returned.
Improving the performance of array queries
Although dealing with arrays in Mongo is relatively efficient out of the box, when dealing with large datasets and complex array queries, performance becomes crucial. For these more demanding circumstances, optimizing queries can significantly reduce execution time and improve your MongoDB application's efficiency and responsiveness. There are some general overlaps with other generic database performance tips, like using indexes, and some that are more specific to MongoDB, like making sure to choose the most efficient operator.
Here are some key strategies to enhance the performance of your array queries:
Indexing
As with most databases, when querying NoSQL data within Mongo, proper indexing is crucial for making sure query execution is efficient. When it comes to indexing, developers should create indexes on the array fields queried most frequently. This allows MongoDB to quickly locate relevant documents without scanning the entire collection.
For example, let's say your database contains a products collection that contains a tags field, holding an array of tags (similar to the examples we used above). If you query the tags array in the products collection frequently, creating an index on tags can significantly improve query performance:
db.products.createIndex({ tags: 1 });
Similarly, if you frequently query on a nested array field, like field.nestedField, within a collection, you can create an index on that field with the following syntax:
db.example.createIndex({ "field.nestedField": 1 });
Query optimization
With indexes in place, another database staple can also be implemented: query optimization. Analyzing your queries and understanding their execution plans is crucial for making sure that queries are performant. One of the best ways to do this is using MongoDB's explain() method, available in both MongoDB Compass and shell. Running this method can provide output that shows valuable insights into how a query is processed, helping a trained eye see how the query could be improved.
For instance, let's consider a query that finds orders containing "Laptop" with a quantity greater than 1:
db.orders.find({ items: { $elemMatch: { product: "Laptop", quantity: { $gt: 1 } } } });
To analyze this query's execution plan, we can use explain() with the "executionStats" verbosity mode set. This is appended to the end of the query:
db.orders.find({ items: { $elemMatch: { product: "Laptop", quantity: { $gt: 1 } } } }).explain("executionStats");
When the query is executed, developers can see an output with detailed information about the query execution, including:
executionTimeMillis. The time taken to execute the query
totalKeysExamined. The number of index keys scanned
totalDocsExamined. The number of documents scanned
stages. The different stages involved in the query execution
By examining these statistics, you can identify potential bottlenecks. Although there could be many outputs depending on the query, let's look at two such outputs from the preceding query. For example:
totalDocsExamined looks higher than expected. This indicates that the query scans many documents during its execution, suggesting a missing or inefficient index. Based on this output, you could potentially solve this by creating an index on the items.product field to speed up the query.
executionTimeMillis is longer than expected. This indicates that the query takes a long time to execute. To solve this, developers could analyze the stages within the query to identify slow operations and optimize them. This could be done by potentially adding indexes or restructuring the query, the details of which will be covered in later points.
Using the output from explain(), developers can boost the performance of their queries by fine-tuning based on the output.
Using efficient operators
Whether it's as you are building the query initially or after you've received feedback from the explain() method, you want choose the most efficient operators for your specific query. For instance, if you only need to check for the existence of an element in an array, a simple match query might be more efficient than using $all or $elemMatch:
// More efficient for checking existencedb.products.find({ tags: "electronics" });// Less efficient for this specific casedb.products.find({ tags: { $all: ["electronics"] } });
Combining knowledge of operators and benchmarking queries, you can find the most efficient operator to use. This will have a massive impact on how performant a query executes.
Limiting data
Unless all of the data in a document is required, generally you want to limit the amount of data being returned by a query. If you only need a subset of the data, use projection to retrieve only the necessary fields. Since this will reduce the amount of data transferred and processed by the query, this should help improve query performance. Here's an example of how you can use projection to only bring back a limited amount of fields, in this case only the name and email fields within a user collection:
// Only retrieve name and emaildb.users.find({ roles: "admin" }, { _id: 0, name: 1, email: 1 });
Avoiding unnecessary array scans
When possible, structure your queries to avoid unnecessary array scans. For example, if you need to check for the presence of a specific value in an array, using $in might be more efficient than using $elemMatch with a single condition:
// More efficientdb.products.find({ tags: { $in: ["electronics"] } });// Less efficientdb.products.find({ tags: { $elemMatch: { $eq: "electronics" } } });
Unwinding and limiting array elements
The aggregation framework provides powerful mechanisms for working with arrays, including unwinding and limiting array elements. To show how the $unwind and $slice operators can be used, let's imagine we have the following data in our database:
db.inventory.insertMany([{ "_id": 1, "item": "ABC", "sizes": ["S", "M", "L"] },{ "_id": 2, "item": "DEF", "sizes": ["M", "L", "XL"] },{ "_id": 3, "item": "GHI", "sizes": ["S", "XL"] }]);
$unwind stage
The $unwind stage is used to deconstruct an array field. The result is that a separate document for each element in the array is created. This is useful for analyzing individual array elements and performing aggregations on them.
Based on the example data, to unwind the sizes array we could do the following:
db.inventory.aggregate([{ $unwind: "$sizes" }]);
This would output:
[{ "_id": 1, "item": "ABC", "sizes": "S" },{ "_id": 1, "item": "ABC", "sizes": "M" },{ "_id": 1, "item": "ABC", "sizes": "L" },{ "_id": 2, "item": "DEF", "sizes": "M" },{ "_id": 2, "item": "DEF", "sizes": "L" },{ "_id": 2, "item": "DEF", "sizes": "XL" },{ "_id": 3, "item": "GHI", "sizes": "S" },{ "_id": 3, "item": "GHI", "sizes": "XL" }]
As you can see in the output, each document now has a single value in the sizes field instead of an array. For specific queries, this can be a more efficient way to process the data than processing the collections with the data as an array.
$slice operator
Another method that can be used is the $slice operator, which can be used within the $project stage to limit the number of elements returned in an array field. This helps control the size of the output and can improve the performance of the query.
For example, based on the example data, to retrieve only the first two sizes for each item, you could execute the following query:
db.inventory.aggregate([{ $project: { _id: 0, item: 1, sizes: { $slice: ["$sizes", 2] } } }]);
This would output the following:
[{ "item": "ABC", "sizes": ["S", "M"] },{ "item": "DEF", "sizes": ["M", "L"] },{ "item": "GHI", "sizes": ["S", "XL"] }]
From this, you could then use the output to perform further queries on the returned data.
Combining $unwind and $slice
In certain scenarios, you may find that you want to unwind a subset of the data, limiting the amount of array entries processed. In this case, you can combine $unwind and $slice to perform operations on a specific portion of an array. For example, once again based on the data, to unwind only the first two sizes for each item in the inventory collection, you would use:
db.inventory.aggregate([{ $project: { _id: 0, item: 1, sizes: { $slice: ["$sizes", 2] } } },{ $unwind: "$sizes" }]);
Advanced array query techniques
Next, let's briefly look at at a few more advanced techniques and examples when it comes to querying and using arrays. Once again, let's imagine that we have the following data set loaded into our database:
db.products.insertMany([{ "_id": 1, "name": "Laptop", "relatedProducts": [2, 3], "tags": ["electronics", "computer", "portable", "work"] },{ "_id": 2, "name": "Mouse", "relatedProducts": [1], "tags": ["electronics", "accessory"] },{ "_id": 3, "name": "Keyboard", "relatedProducts": [1], "tags": ["electronics", "accessory"] }]);
Using this, we will look at how MongoDB offers advanced techniques for querying arrays, allowing you to perform more complex matching and filtering. The following example queries show some more advanced ways to use the skills we've already covered higher up in this blog.
$size operator
The $size operator matches arrays with a specific number of elements. For example, to find products with exactly two tags within the tags array, you would use the following:
db.products.find({ tags: { $size: 2 } });
Based on the data set and this query, we would get the following data returned:
[{"_id": 2,"name": "Mouse","relatedProducts": [1],"tags": ["electronics","accessory"]},{"_id": 3,"name": "Keyboard","relatedProducts": [1],"tags": ["electronics","accessory"]}]
This is because only "Mouse" and "Keyboard" have exactly two tags in the tags array.
$elemMatch with complex criteria
You can use $elemMatch with more complex criteria, including nested comparisons and logical operators. For example, to find products with a tag that starts with "electr" and another tag that ends with "able", you would use:
db.products.find({tags: {$all: [{ $elemMatch: { $regex: /^electr/ } },{ $elemMatch: { $regex: /able$/ } }]}});
This would return:
[{"_id": 1,"name": "Laptop","relatedProducts": [2,3],"tags": ["electronics","computer","portable","work"]}]
This is because only "Laptop" has tags that both start with "electr" ("electronics") and end with "able" ("portable").
Aggregation framework for advanced filtering
The aggregation framework provides powerful tools for filtering array elements. You can use $match, $unwind, $group and other stages to filter, transform and aggregate array data.
For example, to find products with at least two tags that start with "e", you could use:
db.products.aggregate([{ $unwind: "$tags" },{ $match: { tags: { $regex: /^e/ } } },{ $group: { _id: "$_id", count: { $sum: 1 } } },{ $match: { count: { $gte: 1 } } }]);
This would return:
[{ _id: 3, count: 1 },{ _id: 1, count: 1 },{ _id: 2, count: 1 }]
This is because "Laptop", "Mouse" and "Keyboard" have at least one tag in its tags array that starts with the letter "e".
Boosting array Performance with SingleStore Kai™️
Do you need a performance boost for your MongoDB array queries? SingleStore Kai, a MongoDB-compatible API, can significantly accelerate your workloads.
Here's how SingleStore Kai enhances array handling:
Blazing-fast execution. Experience up to 100x faster query execution for complex array operations, thanks to SingleStore Kai's optimized architecture and vectorized processing.
Improved indexing. Leverage array indexes and other advanced indexing options for enhanced performance.
Effortless scalability. Handle massive datasets and high query volumes with ease.
SQL integration. Combine MongoDB's array operators with the power of SQL for advanced analysis.
Ready to supercharge your array queries? Migrate your MongoDB workloads to SingleStore Kai and unlock new levels of performance and scalability.
Try SingleStore free
Mastering MongoDB array queries is essential for efficiently retrieving and manipulating data in your NoSQL applications. By understanding the various methods and operators available, you can effectively navigate and filter array elements — even within complex nested documents. Remember, a crucial component of creating queries is to also optimize them with proper indexing and efficient operators. This helps to ensure optimal performance, especially when dealing with large datasets that generally tend to bog down such queries.
Of course, if you want to experience a significant boost in your MongoDB array query performance, you can always try a free trial of SingleStore Kai. With SingleStore Kai, developers can unlock the power of a distributed SQL database with a MongoDB-compatible API. It delivers blazing-fast speed, effortless scalability, and seamless integration with your existing MongoDB tools and workflows. Try it out for yourself today and see the difference in flexibility and performance!